Introduction to Python Python is an interpreted language. It means that Python directly executes the code line by line. It converts the source code that we write into an intermediate language, ...
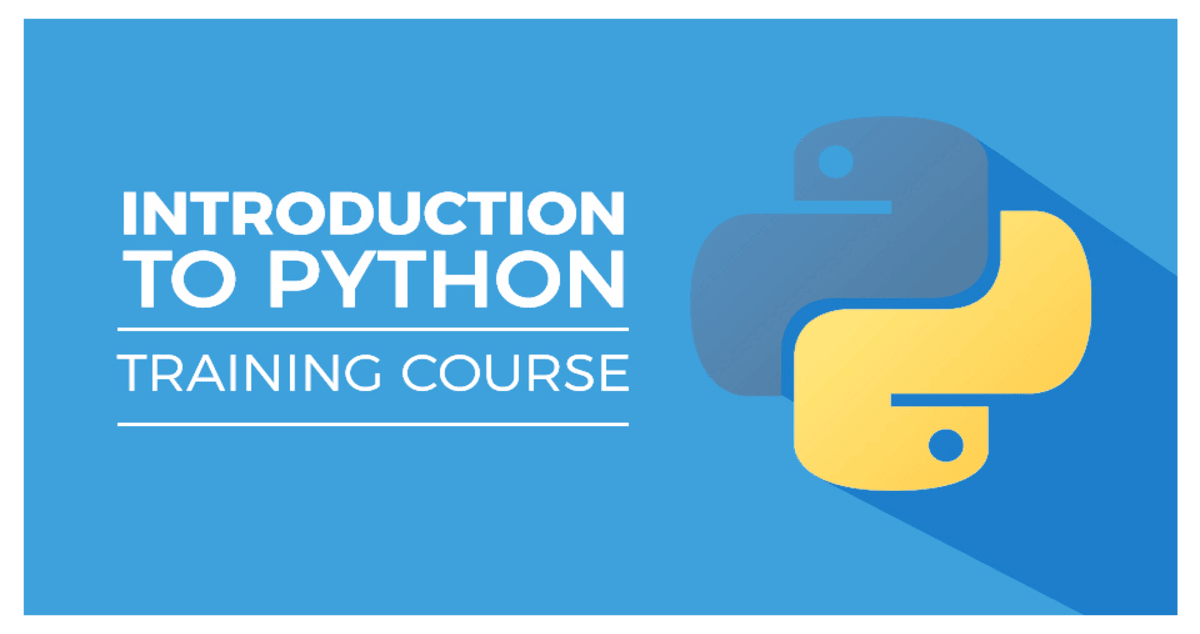
01. Introduction to Python
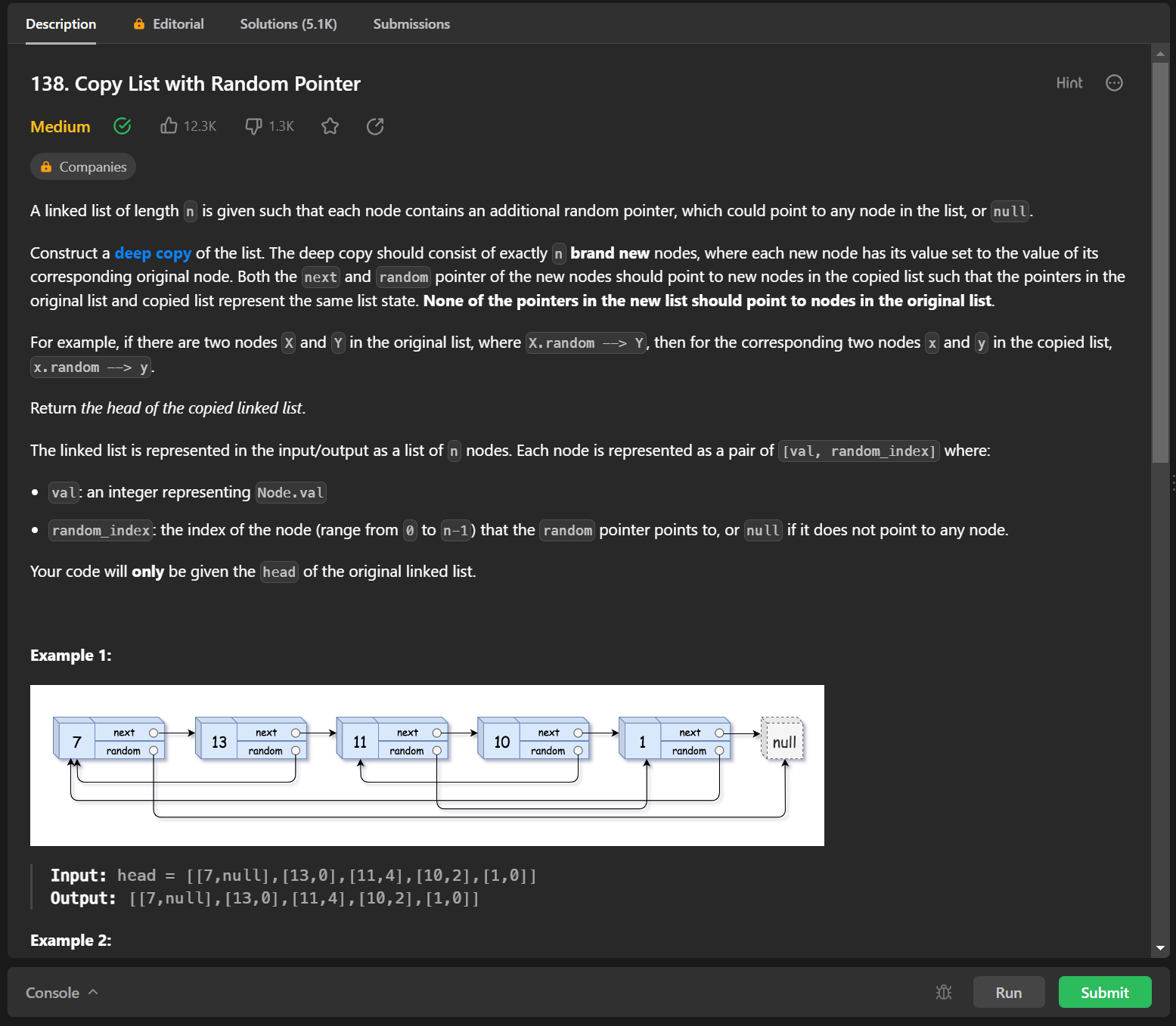
138. Copy List with Random Pointer
Go to the Problem 138. Copy List with Random Pointer Solution Approach 1: Recursive with O(N) Space In this approach, we take advantage of the fact that we already have a hash map containing ma...
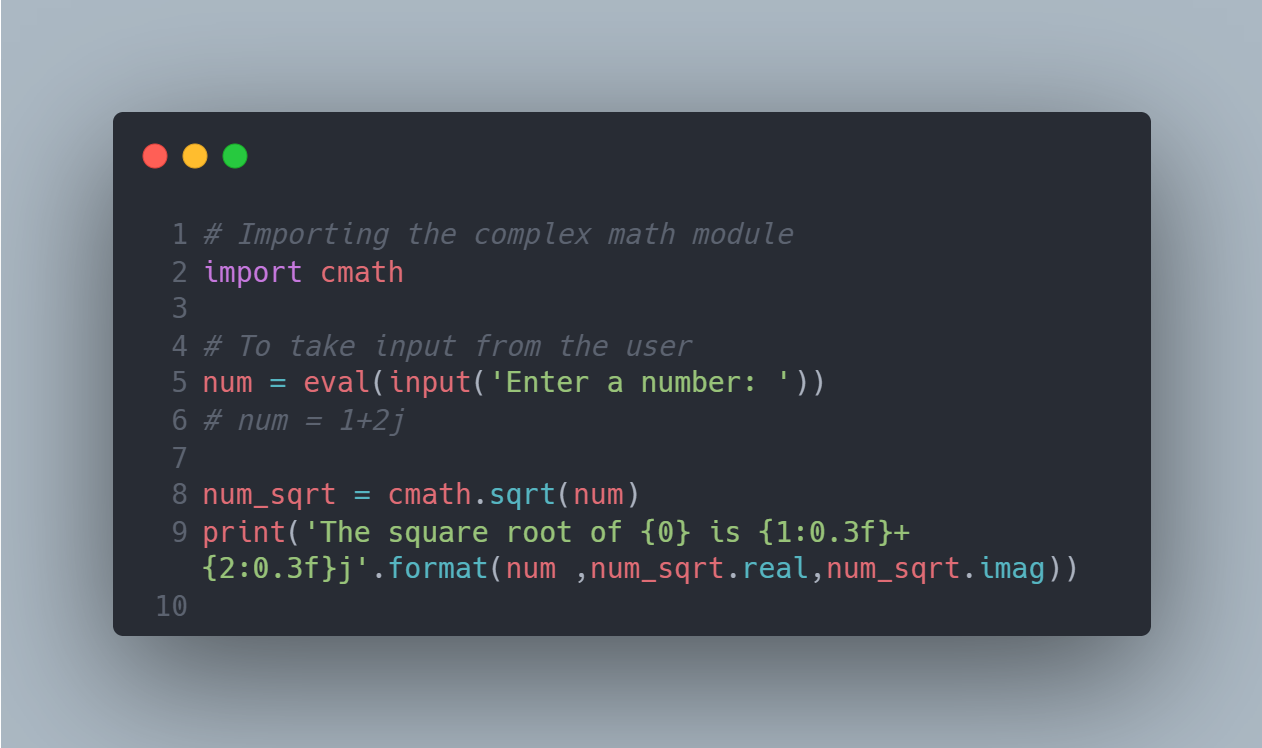
03. Find Square Root
Ex 1: For Positive Numbers # Python Program to calculate the square root # Note: change this value for a different result num = 8 # uncomment to take the input from the user #num = float(input('...
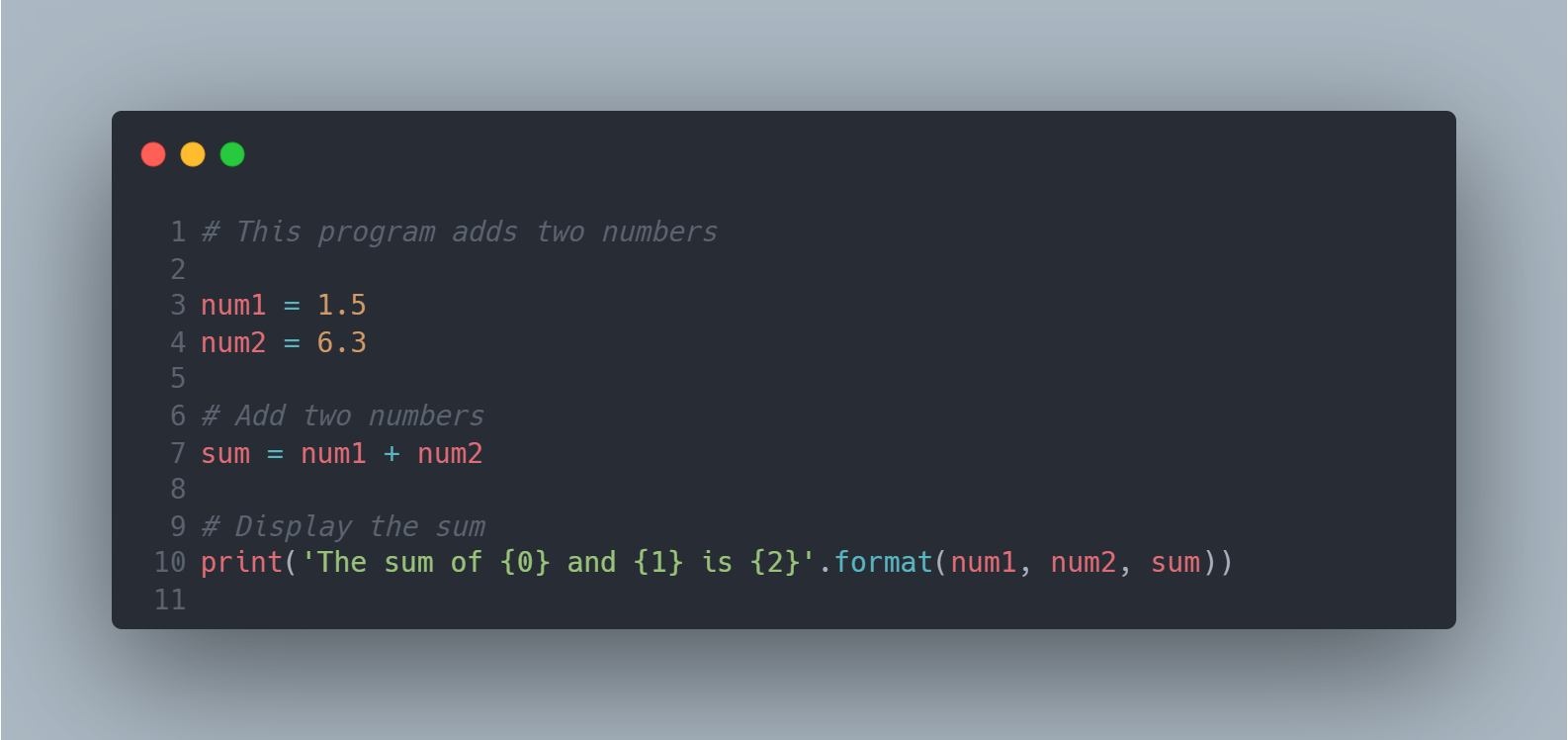
02. Add Two Numbers
Example 1: Add Two Numbers Program # This program adds two numbers num1 = 1.5 num2 = 6.3 # Add two numbers sum = num1 + num2 # Display the sum print(f'The sum of {num1} and {num2} is {sum}') ...
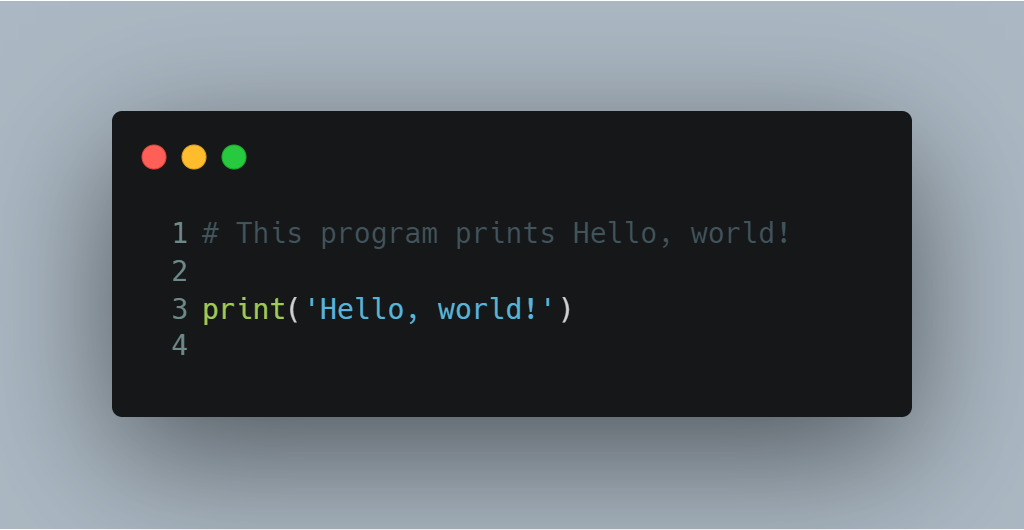
01. Print "Hello World!"
1. Hello World Program # This program prints Hello World! on the screen print("Hello World!") In this program, we have used the built-in print() function to print the string Hello, world! on our...
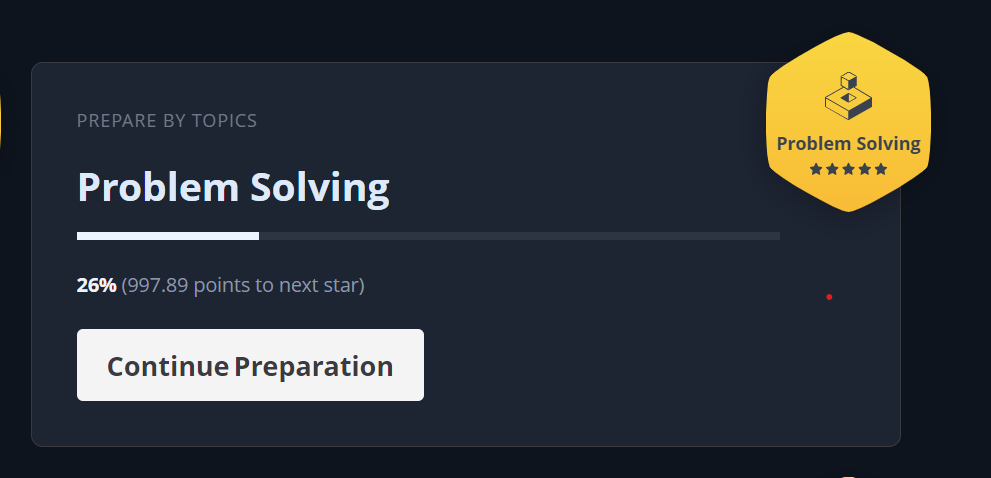
02. Dynamic Arrays
Problem: Dynamic Arrays Solution 1: Using for loop and if-else statement def dynamicArray(n, queries): seqList = [[] for _ in range(n)] lastAnswer = 0 result = [] for query in que...
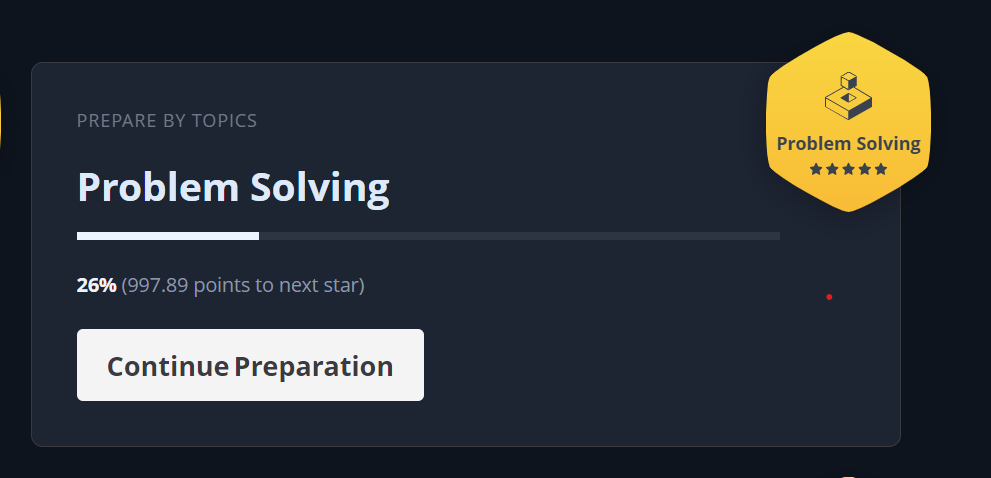
Learn Data Science step by step
Introduction to Data Science Data Science Importance is growing day by day. Data Science is a combination of various tools, algorithms, and machine learning principles with the goal to discover hi...
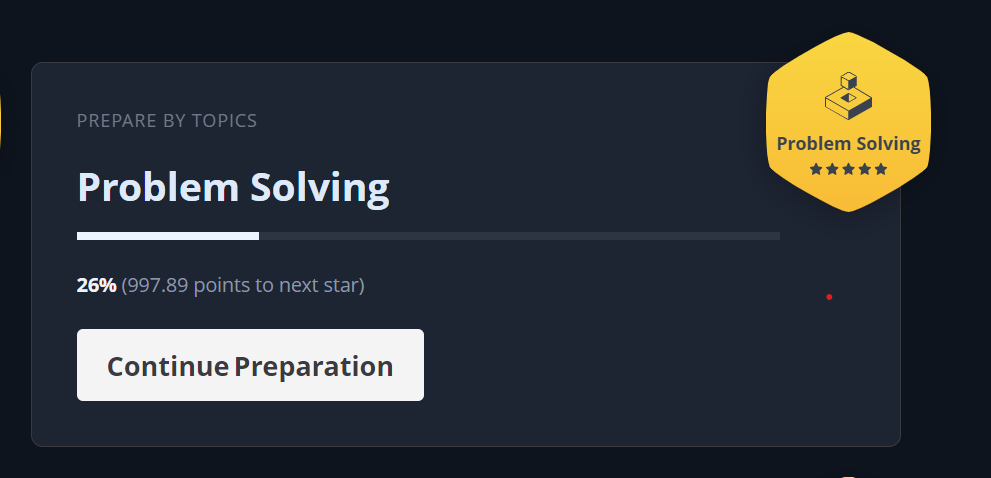
01. Arrays - DS
Problem: Arrays - DS Solution 1: Using for loop and storing in another array def reverseArray(a): arr = [] for i in range(len(a) - 1, -1, -1): arr.append(a[i]) return arr Sol...
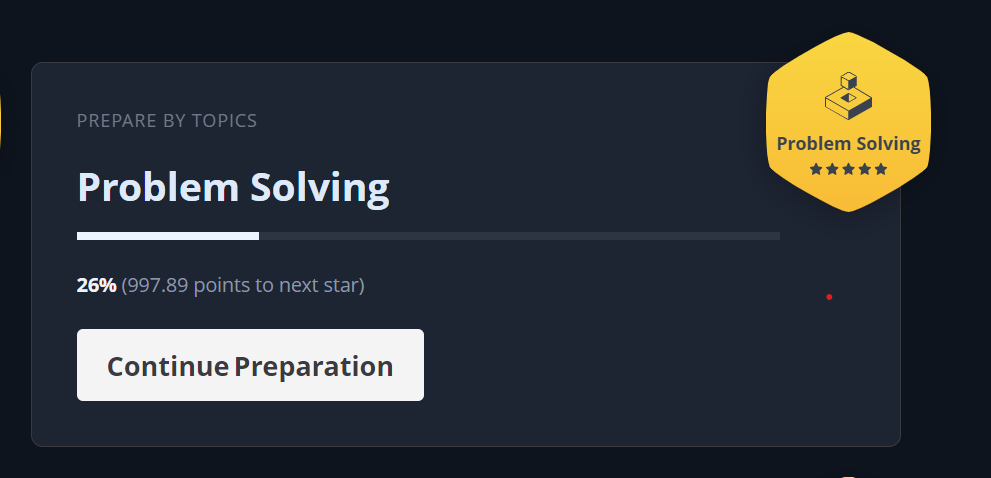
1. Introduction to Linked List
1. Introduction to linked list What is Linked List Like arrays, a Linked List is a linear data structure. Unlike arrays, linked list elements are not stored at a contiguous location; the elements...